Apply suggestions from code review
Co-authored-by: Robert Jördens <rj@quartiq.de>
This commit is contained in:
parent
d6dfbd2c88
commit
f67a56c2cd
|
@ -25,28 +25,30 @@ which allows for a wide variety of experimental uses, such as digital filter des
|
|||
implementation of digital lockin schemes.
|
||||
|
||||
This documentation is intended to bring a user up to speed on using Stabilizer and the firmware
|
||||
provided by Quartiq.
|
||||
provided by QUARTIQ and contributors.
|
||||
|
||||
## Hardware
|
||||
|
||||
The Stabilizer hardware is managed via a [separate repository](https://github.com/sinara-hw/Stabilizer).
|
||||
Some information about the hardware is gathered in the [Stabilizer wiki](https://github.com/sinara-hw/Stabilizer/wiki). More detailed data, measurements, discussions, and tests have been posted in the [Stabilizer issue tracker](https://github.com/sinara-hw/Stabilizer/issues?q=is%3Aissue).
|
||||
|
||||
[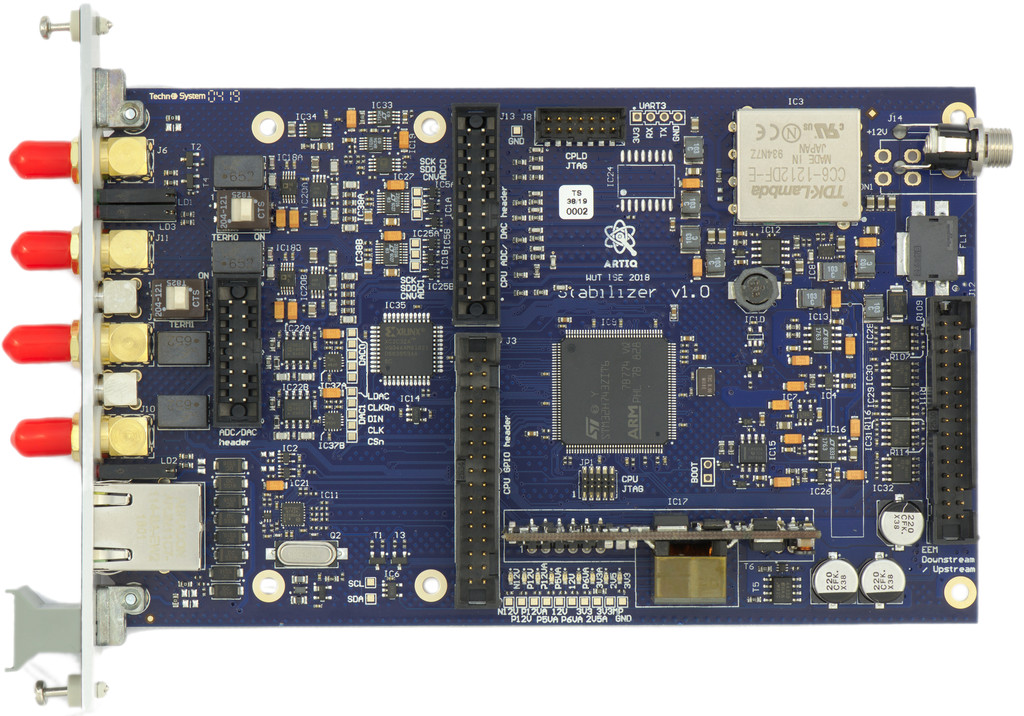](https://github.com/sinara-hw/Stabilizer)
|
||||
|
||||
Stabilizer can be extended and coupled with a mezzanine board. One such mezzanine is the DDS upconversion/downconversion frontend Pounder. The Pounder hardware is managed via a [separate repository](https://github.com/sinara-hw/Pounder), again with [wiki](https://github.com/sinara-hw/Pounder/wiki) and [issue tracker](https://github.com/sinara-hw/Pounder/issues?q=is%3Aissue).
|
||||
## Applications
|
||||
|
||||
This firmware offers a library of hardware and software functionality targeting the use of the Stabilizer hardware in various digital signal processing applications commonly occurring in Quantum Technology.
|
||||
It provides abstractions over the fast analog inputs and outputs, time stamping, Pounder DDS interfaces and a collection of tailored and optimized digital signal processing algorithms (IIR, FIR, Lockin, PLL, reciprocal PLL, Unwrapper, Lowpass, Cosine-Sine, Atan2).
|
||||
It provides abstractions over the fast analog inputs and outputs, time stamping, Pounder DDS interfaces and a collection of tailored and optimized digital signal processing algorithms (IIR, FIR, Lockin, PLL, reciprocal PLL, Unwrapper, Lowpass, Cosine-Sine, Atan2) in the [DSP crate]({{site.baseurl}}/firmware/dsp/index.html).
|
||||
An application, which is the compiled firmware running on the device, can compose and configure these hardware and software components to implement different use cases.
|
||||
Several applications are provided by default.
|
||||
|
||||
The following documentation links contain the application-specific settings and telemetry
|
||||
information.
|
||||
|
||||
| Application | Documentation | Application Description |
|
||||
| :---: | :--: | :---- |
|
||||
| `dual-iir` | [Link]({{site.baseurl}}/firmware/dual_iir/index.html) | Two channel biquad IIR filter
|
||||
| `lockin` | [Link]({{site.baseurl}}/firmware/lockin/index.html) | Lockin amplifier support various various reference sources |
|
||||
| Application | Description |
|
||||
| :---: | :---- |
|
||||
| [`dual-iir`]({{site.baseurl}}/firmware/dual_iir/index.html) | Two channel biquad IIR filter |
|
||||
| [`lockin`]({{site.baseurl}}/firmware/lockin/index.html) | Lockin amplifier support various various reference sources |
|
||||
|
||||
### Library Documentation
|
||||
The Stabilizer library docs contain documentation for common components used in all Stabilizer
|
||||
|
|
|
@ -14,18 +14,11 @@ nav_order: 2
|
|||
|
||||
# Getting Started
|
||||
|
||||
Getting started requires a few steps:
|
||||
There are a number of steps that must be completed when first getting started with Stabilizer.
|
||||
1. Configure the firmware
|
||||
* This requires updating any parameters, such as static IP addresses and
|
||||
sampling rate.
|
||||
1. Build the application
|
||||
* This requires compiling the code after configuration parameters have been
|
||||
updated.
|
||||
1. Upload the application
|
||||
* Once fimrware has been built, it needs to be programmed onto the device.
|
||||
1. Set up MQTT
|
||||
* Stabilizer utilizes MQTT for telemetry and configuration.
|
||||
1. Set parameters in the firmware source code, such as IP addresses and sampling rate.
|
||||
1. Build the application by compiling the source code.
|
||||
1. Upload the application and programming it onto the device.
|
||||
1. Set up MQTT for telemetry and configuration.
|
||||
|
||||
The following sections will walk you through completing each of these steps.
|
||||
|
||||
|
@ -40,11 +33,11 @@ desired application.
|
|||
|
||||
Stabilizer firmware contains compile-time parameters that may need to be changed based on
|
||||
application requirements. Some examples of parameters that may require configuraton:
|
||||
* Sample frequency
|
||||
* Sample batch size
|
||||
* Sampling interval.
|
||||
* Batch size.
|
||||
* MQTT Broker IP address
|
||||
|
||||
Parameters are configured by editing `src/configuration.rs`.
|
||||
Parameters are configured by editing the file `src/configuration.rs`.
|
||||
|
||||
Refer to the [documentation]({{site.baseurl}}/firmware/stabilizer/configuration/index.html) for more
|
||||
information on parameters.
|
||||
|
|
|
@ -42,7 +42,7 @@ pub type Vec5 = [f32; 5];
|
|||
///
|
||||
/// # Miniconf
|
||||
///
|
||||
/// `{"y_offset": y0, "y_min": ym, "y_max": yM, "ba": [b0, b1, b2, -a1, -a2]}`
|
||||
/// `{"y_offset": y_offset, "y_min": y_min, "y_max": y_max, "ba": [b0, b1, b2, a1, a2]}`
|
||||
///
|
||||
/// * `y0` is the output offset code
|
||||
/// * `ym` is the lower saturation limit
|
||||
|
@ -52,12 +52,6 @@ pub type Vec5 = [f32; 5];
|
|||
/// new output is computed as `y0 = a1*y1 + a2*y2 + b0*x0 + b1*x1 + b2*x2`.
|
||||
/// The IIR coefficients can be mapped to other transfer function
|
||||
/// representations, for example as described in <https://arxiv.org/abs/1508.06319>
|
||||
///
|
||||
///
|
||||
/// ## Notes
|
||||
/// The units of the IIR utilize 16-bit signed integers for full-scale. saturation and offset
|
||||
/// parameter are given in this scale, where full-scale represents an output amplitude of 10.24
|
||||
/// V.
|
||||
#[derive(Copy, Clone, Debug, Default, Deserialize, MiniconfAtomic)]
|
||||
pub struct IIR {
|
||||
pub ba: Vec5,
|
||||
|
|
|
@ -11,7 +11,7 @@ pub const MQTT_BROKER: [u8; 4] = [10, 34, 16, 10];
|
|||
///
|
||||
/// # Units
|
||||
/// The units of this parameter are specified as a logarithmic number of ticks of the internal
|
||||
/// timer, which runs at 100MHz.
|
||||
/// timer, which runs at 100 MHz.
|
||||
///
|
||||
/// ## Example
|
||||
/// With a value of 7, this corresponds to 2^7 = 128 ticks. Each tick of the 100MHz timer requires
|
||||
|
|
Loading…
Reference in New Issue